Fixing an old dehumidifier with Arduino
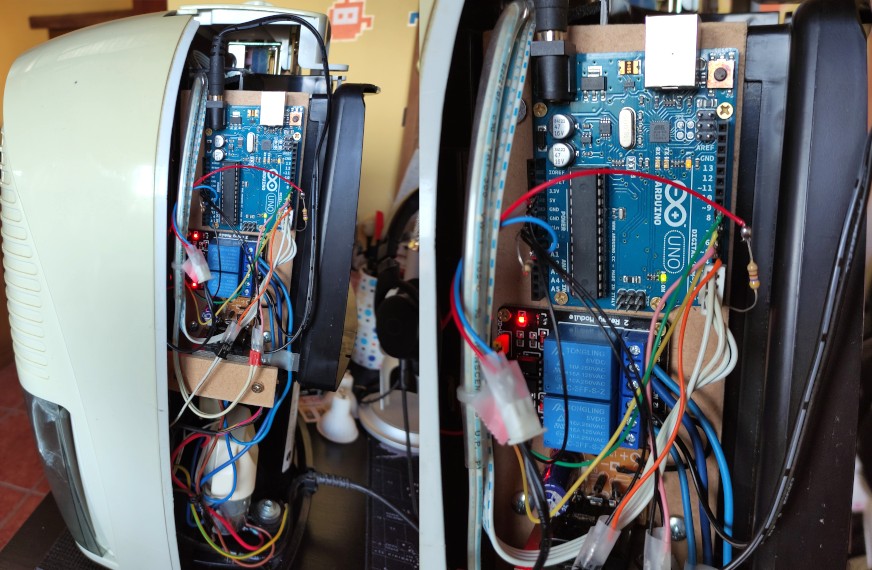
I purchased an Arduino UNO board approximately 10 years ago and conducted various experiments with it. Recently, my old Delonghi DEM 10 dehumidifier ceased to function due to a board issue, and the cost of replacing the original board was approximately 60€. Instead, I opted to replace the faulty board with the Arduino UNO and a two-relay board (designated for the compressor and fan).
BOM:
- Arduino UNO: 20€
- Relay board: 5€
- 12V transformer: found at home
- ~1€ in cables and screws
- A resistor
- Wood recycled from a packaging
And a new dehumidifier with the same specs costs ~150€, but I didn’t do this for the money….
I had a lot of fun coding the program to manage the sensors and the timer. I remembered how thermistors work and implemented the defrost and the overheat protection with a state machine, repurposing the leds to show the states. After stopping the compressor we need to wait ~20 seconds before starting it again (because the capacitor needs to be loaded).
This is the source code, it uses the thermistor library.:
#include "thermistor.h"
const int STATUS_IDLE = 0;
const int STATUS_WORKING = 1;
const int STATUS_PAUSE = 2;
const int PIN_FULL = 6;
const int PIN_HIGRO = 7;
const int PIN_COMP = 8;
const int PIN_VENT = 9;
const int PIN_THERMISTOR = A0;
const int PIN_LED_ON = 4;
const int PIN_LED_DEFROST = 3;
const int PIN_LED_PAUSE = 2;
const long TIME_WAIT = 50;
const long TIME_BEFORE_PAUSE = 25 * 60 * 1000L;
const long TIME_PAUSE_TIMER = 5 * 60 * 1000L;
const long TIME_PAUSE_DEFROST = 10 * 60 * 1000L;
const long TIME_PAUSE_OVERHEAT = 10 * 60 * 1000L;
const long TIME_PAUSE_COMP_OFF = 30 * 1000L;
const int TEMP_DEFROST = 30; // IN 1/10 ºC
const int TEMP_OVERHEAT = 350; // IN 1/10 ºC
int status = STATUS_PAUSE;
long workingTimer = TIME_BEFORE_PAUSE;
long pauseTimer = TIME_PAUSE_COMP_OFF;
THERMISTOR thermistor(PIN_THERMISTOR,
10000, // Nominal resistance at 25 ºC
3950, // thermistor's beta coefficient
10000); // Value of the series resistor
void setup() {
Serial.begin(9600);
pinMode(PIN_FULL, INPUT_PULLUP);
pinMode(PIN_HIGRO, INPUT_PULLUP);
pinMode(PIN_COMP, OUTPUT);
pinMode(PIN_VENT, OUTPUT);
digitalWrite(PIN_VENT, HIGH);
digitalWrite(PIN_COMP, HIGH);
pinMode(PIN_LED_ON, OUTPUT);
pinMode(PIN_LED_DEFROST, OUTPUT);
pinMode(PIN_LED_PAUSE, OUTPUT);
digitalWrite(PIN_LED_ON, HIGH);
digitalWrite(PIN_LED_DEFROST, HIGH);
digitalWrite(PIN_LED_PAUSE, HIGH);
}
void loop() {
long t1 = millis();
boolean full = digitalRead(PIN_FULL);
boolean higroOff = digitalRead(PIN_HIGRO);
uint16_t temp = thermistor.read();
// Status changes
switch(status) {
case STATUS_WORKING: {
if (full || higroOff) {
Serial.println("Stopping...");
status = STATUS_PAUSE;
pauseTimer = TIME_PAUSE_COMP_OFF;
} else if (temp < TEMP_DEFROST) {
Serial.println("Pausing to defrost...");
status = STATUS_PAUSE;
pauseTimer = TIME_PAUSE_DEFROST;
} else if (temp > TEMP_OVERHEAT) {
Serial.println("Pausing due to overheat ...");
status = STATUS_PAUSE;
pauseTimer = TIME_PAUSE_OVERHEAT;
} else if (workingTimer <= 0) {
Serial.println("Pausing due to timer...");
workingTimer = TIME_BEFORE_PAUSE;
status = STATUS_PAUSE;
pauseTimer = TIME_PAUSE_TIMER;
}
break;
}
case STATUS_IDLE: {
if (!full && !higroOff) {
Serial.println("Starting...");
status = STATUS_WORKING;
}
break;
}
case STATUS_PAUSE: {
if (pauseTimer <= 0) {
status = STATUS_IDLE;
}
break;
}
}
// New statuses and timer update
switch(status) {
case STATUS_WORKING: {
Serial.println("Working");
digitalWrite(PIN_VENT, LOW);
digitalWrite(PIN_COMP, LOW);
digitalWrite(PIN_LED_ON, LOW);
digitalWrite(PIN_LED_DEFROST, HIGH);
digitalWrite(PIN_LED_PAUSE, HIGH);
break;
}
case STATUS_IDLE: {
Serial.println("Idle");
digitalWrite(PIN_VENT, HIGH);
digitalWrite(PIN_COMP, HIGH);
digitalWrite(PIN_LED_ON, HIGH);
digitalWrite(PIN_LED_DEFROST, LOW);
digitalWrite(PIN_LED_PAUSE, HIGH);
break;
}
case STATUS_PAUSE: {
Serial.println("Paused");
digitalWrite(PIN_VENT, LOW);
digitalWrite(PIN_COMP, HIGH);
digitalWrite(PIN_LED_ON, HIGH);
digitalWrite(PIN_LED_DEFROST, HIGH);
digitalWrite(PIN_LED_PAUSE, LOW);
break;
}
}
// Pause and timer updates
Serial.print("Temp in 1/10 ºC : ");
Serial.println(temp);
delay(TIME_WAIT);
long t2 = millis();
switch(status) {
case STATUS_WORKING: {
workingTimer -= t2 - t1;
Serial.println(workingTimer);
break;
}
case STATUS_PAUSE: {
pauseTimer -= t2 - t1;
Serial.println(pauseTimer);
break;
}
}
}
And it feels like if I do not suck at electronics anymore 🙂