Using styles on Android layouts
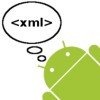
A very cool feature of Android that many developers do not know (at least me 6 months ago) is the possibility of using “styles” on Android XML layouts. This thing is similar to CCS on HTML and provides a method to simplify the XML design.
First, let’s see an example of a XML layout without styles, you can notice the need to repeat attributes and the difficulty of reading the XML:
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:text="@string/text1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textStyle="bold"
android:textColor="@color/white"
/>
<TextView
android:text="@string/text2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textStyle="bold"
android:textColor="@color/red"
/>
</LinearLayout>
But we can define styles grouping this attributes on the file res/values/styles.xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
<style name="VerticalLinearLayout">
<item name="android:layout_width">wrap_content</item>
<item name="android:layout_height">wrap_content</item>
<item name="android:orientation">vertical</item>
</style>
<style name="WhiteText">
<item name="android:layout_width">wrap_content</item>
<item name="android:layout_height">wrap_content</item>
<item name="android:orientation">vertical</item>
<item name="android:textStyle">bold</item>
<item name="android:textColor">@color/white</item>
</style>
<style name="RedText" parent="@style/WhiteText">
<item name="android:textColor">@color/red</item>
</style>
</resources>
Note te possibility of inheriting styles, in this example “RedText” is inherited from the “WhiteText” style changing only the text color.
Our XML layout, with styles, gets reduced to:
<LinearLayout style="@style/VerticalLinearLayout"/>
<TextView style="@style/WhiteText"
android:text="@string/text1"
/>
<TextView style="@style/RedText"
android:text="@string/text2"
/>
</LinearLayout>
Finally, you can see that I’m using colors (@color/white and @color/red) which must be defined on the file res/values/colors.xml (in a similar way to strings):
<?xml version="1.0" encoding="utf-8"?>
<resources>
<color name="white">#ffffffff</color>
<color name="red">#ffff0000</color>
</resources>